Convert Date to Timestamp in PHP: Comprehensive Guide
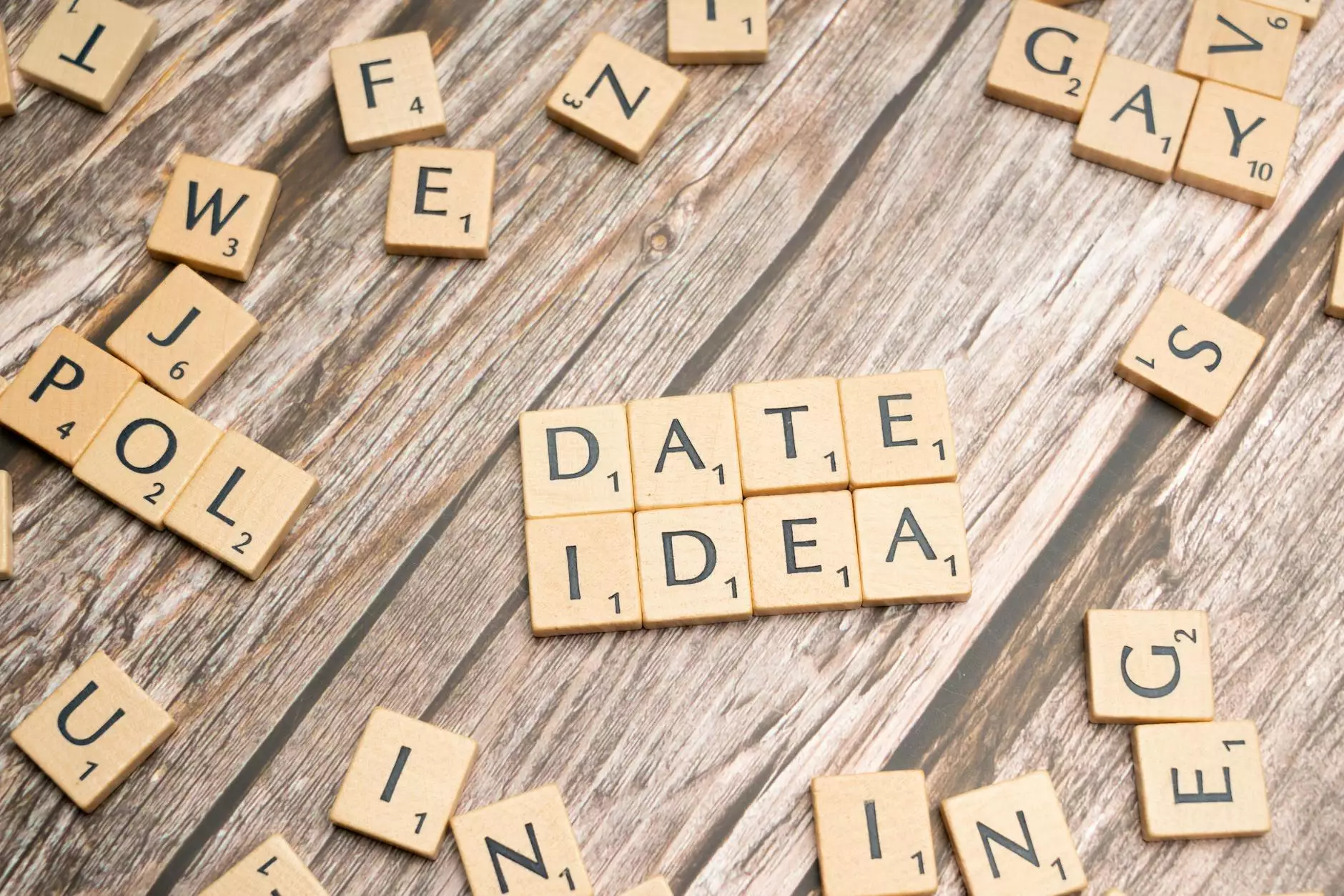
In the realm of web development, PHP is widely recognized for its versatility and ease of use. One common requirement when working with dates is the ability to convert date to timestamp in PHP. This process is crucial for various applications, such as database operations, time zone manipulations, and scheduling tasks. In this article, we will delve into the detailed steps, methods, and best practices for achieving this task effectively.
Understanding Timestamps and Their Importance
A timestamp is a numerical representation of a specific date and time, measured in seconds since January 1, 1970 (the Unix Epoch). This format is essential in computing because it provides a standardized way to record and compare dates and times across different systems. Here are a few key points about timestamps:
- Uniformity: Timestamps offer a consistent format regardless of the user's locale or timezone.
- Ease of Calculation: Performing date calculations (like getting the difference between two dates) becomes significantly easier with timestamps.
- Compatibility: Most databases and programming languages support Unix timestamps, making data interchange more seamless.
Why Use PHP for Date Conversion?
PHP offers a robust set of functions for date manipulation and conversion. Its built-in capabilities allow developers to easily handle dates and times, making it a preferred choice for web applications. Here’s why using PHP for the conversion of dates to timestamps is advantageous:
- Simplicity: PHP provides easy-to-use functions like strtotime() and DateTime for effective conversion.
- Flexibility: PHP allows for the parsing of various date formats, which is crucial for applications that rely on user input.
- Community Support: Given PHP's popularity, there is vast documentation and community support available for resolving any issues that arise.
How to Convert Date to Timestamp Using PHP
Method 1: Using `strtotime()` Function
The simplest way to convert a date to a timestamp in PHP is by using the strtotime() function. This function takes a date string as input and returns the corresponding Unix timestamp.
In the example above, the date string is converted into a timestamp. It’s important to note that the default timezone of your PHP environment will affect how some dates are processed. Therefore, it’s advisable to set the appropriate timezone using the date_default_timezone_set() function:
Method 2: Using `DateTime` Class
The DateTime class is a powerful alternative that offers more flexibility and functionality when dealing with dates and timestamps. Here’s how you can use it to achieve the same conversion:
The DateTime class is particularly useful for complex date manipulations, including adding or subtracting periods and formatting outputs. Let’s explore how to format a timestamp:
Handling Different Date Formats
One of the challenges developers face is dealing with different date formats provided by users or external sources. Fortunately, PHP is capable of parsing various formats without extensive manual intervention.
Using `DateTime::createFromFormat()`
The DateTime::createFromFormat() method allows you to specify the format of the input date string, making it easier to parse custom formats:
Best Practices for Date and Time Handling in PHP
When dealing with date conversion, following best practices ensures that your application remains robust and error-free. Here are some valuable tips:
- Always Set the Time Zone: To avoid unexpected results, always set the correct timezone for your application using date_default_timezone_set().
- Validate User Input: Always validate and sanitize user input before processing dates to prevent errors and security issues.
- Be Aware of Edge Cases: Consider how leap years, daylight saving time, and different calendar systems can affect your date calculations.
- Use Exception Handling: Implement error handling when working with date and time to manage potential issues gracefully.
Common Use Cases for Date to Timestamp Conversion
Understanding the practical applications of converting date to timestamp in PHP can enhance your programming skills. Here are some common scenarios:
1. Storing Dates in a Database
Most databases, such as MySQL, require dates to be stored in specific formats. Converting dates to timestamps can help ensure consistency and ease of retrieval when querying date-related information.
2. Scheduling Tasks
In applications where tasks are scheduled (such as cron jobs), timestamps are often necessary to determine the exact time a task should execute.
3. Logging Events
When logging events in applications, timestamps give you a precise record of when each event occurred, which is crucial for debugging and auditing.
Conclusion
In summary, converting date to timestamp in PHP is a fundamental skill that every web developer should master. Utilizing PHP's built-in functions and classes allows you to handle dates efficiently, providing benefits in terms of application performance and accuracy. By following the guidelines and best practices outlined in this comprehensive guide, you can enhance your coding abilities and ensure your applications effectively manage dates and times.
Whether you are working in web design or software development, mastering date manipulation will open doors to developing more complex and functional applications. Start practicing today and elevate your PHP programming expertise!
convert date to timestamp php